Getting Started with Next.js: Structure, Tips & Deployment
By Muhammad Sharjeel | Published on 2025-04-13
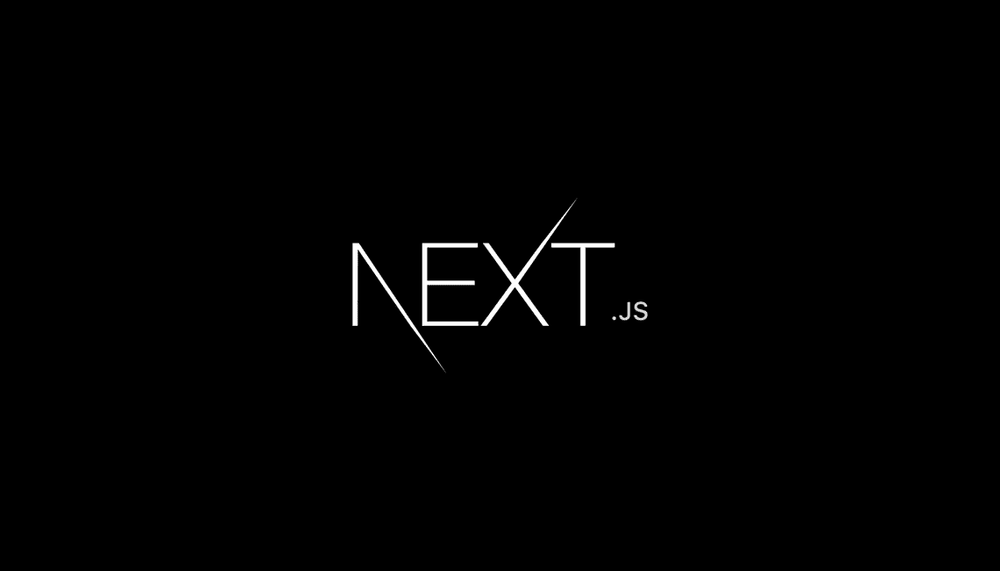
What is Next.js?
Next.js is a powerful React-based framework that enables developers to build fast, SEO-friendly web applications with minimal setup. Whether you're building a static marketing page, a full-stack SaaS product, or a blog — Next.js handles routing, rendering modes, and performance out of the box.
Why Choose Next.js?
- File-based routing: No need for React Router. Just drop a file in
/app
or/pages
and it becomes a route. - Server-side rendering & static generation: Easily switch between SSR, SSG, or client-side rendering.
- Built-in API routes: Create full-stack apps without needing a separate backend.
- Seamless Vercel deployment: Optimized for Vercel hosting.
Project Setup
Let’s create a new Next.js app using the App Router approach (recommended in Next.js 13+).
npx create-next-app@latest my-nextjs-app --experimental-app
After the CLI prompts, your project will be initialized with the following folder structure:
Project Structure Overview
.
├── app/ // App Router entrypoint
│ ├── layout.tsx // Root layout (wrapping component)
│ ├── page.tsx // Homepage ("/")
│ └── (routes)/ // Sub-routes as directories
├── components/ // Shared UI components
├── public/ // Static assets (e.g. images, fonts)
├── styles/ // Tailwind/global styles
├── lib/ // Utilities, helper functions
├── .env.local // Environment variables
├── tailwind.config.ts // Tailwind configuration
├── next.config.js // Framework-level config
└── package.json
This modular layout promotes separation of concerns and scalability.
Adding Tailwind CSS
Tailwind helps you build modern UIs rapidly. To install it:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Update your tailwind.config.ts
to include:
content: ["./app/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}"]
And in your global CSS file (usually globals.css
):
@tailwind base;
@tailwind components;
@tailwind utilities;
Routing with the App Router
Next.js App Router introduces nested layouts, dynamic routing, and server components by default.
Create a route:
// app/about/page.tsx
export default function AboutPage() {
return <h1>About Me</h1>
}
This will now be available at /about
.
Nested Layouts:
// app/dashboard/layout.tsx
export default function DashboardLayout({ children }) {
return (
<div>
<Sidebar />
{children}
</div>
)
}
This layout wraps all routes under /dashboard
.
Environment Configuration
Create a .env.local
file in your root for environment-specific settings:
NEXT_PUBLIC_API_BASE_URL=https://api.example.com
NEXT_PUBLIC_ENV=development
Access these with process.env.NEXT_PUBLIC_*
in your codebase.
Useful Libraries to Add
- clsx / tailwind-merge – Handle conditional classNames.
- shadcn/ui – Prebuilt headless UI components with Tailwind.
- framer-motion – For page transitions and motion effects.
- zod – For schema validation in forms or APIs.
Deploying to Vercel (Free Hosting)
- Push your code to GitHub.
- Go to vercel.com and sign in.
- Click “New Project” and import your GitHub repo.
- Vercel auto-detects the framework and deploys your app instantly.
Bonus: You get previews on every commit and custom domains. You can also add environment variables from the Vercel dashboard.
Final Thoughts
Next.js offers a scalable foundation whether you're just starting out or building enterprise-level applications. Understanding the project structure and deployment process will help you ship better, faster.
If you're new to the ecosystem, start small — build a portfolio, a blog, or a dashboard UI. The tooling is designed to grow with you.