Using Docker & NGINX to Build a Suite of Microservices
By Muhammad Sharjeel | Published on 2025-04-13
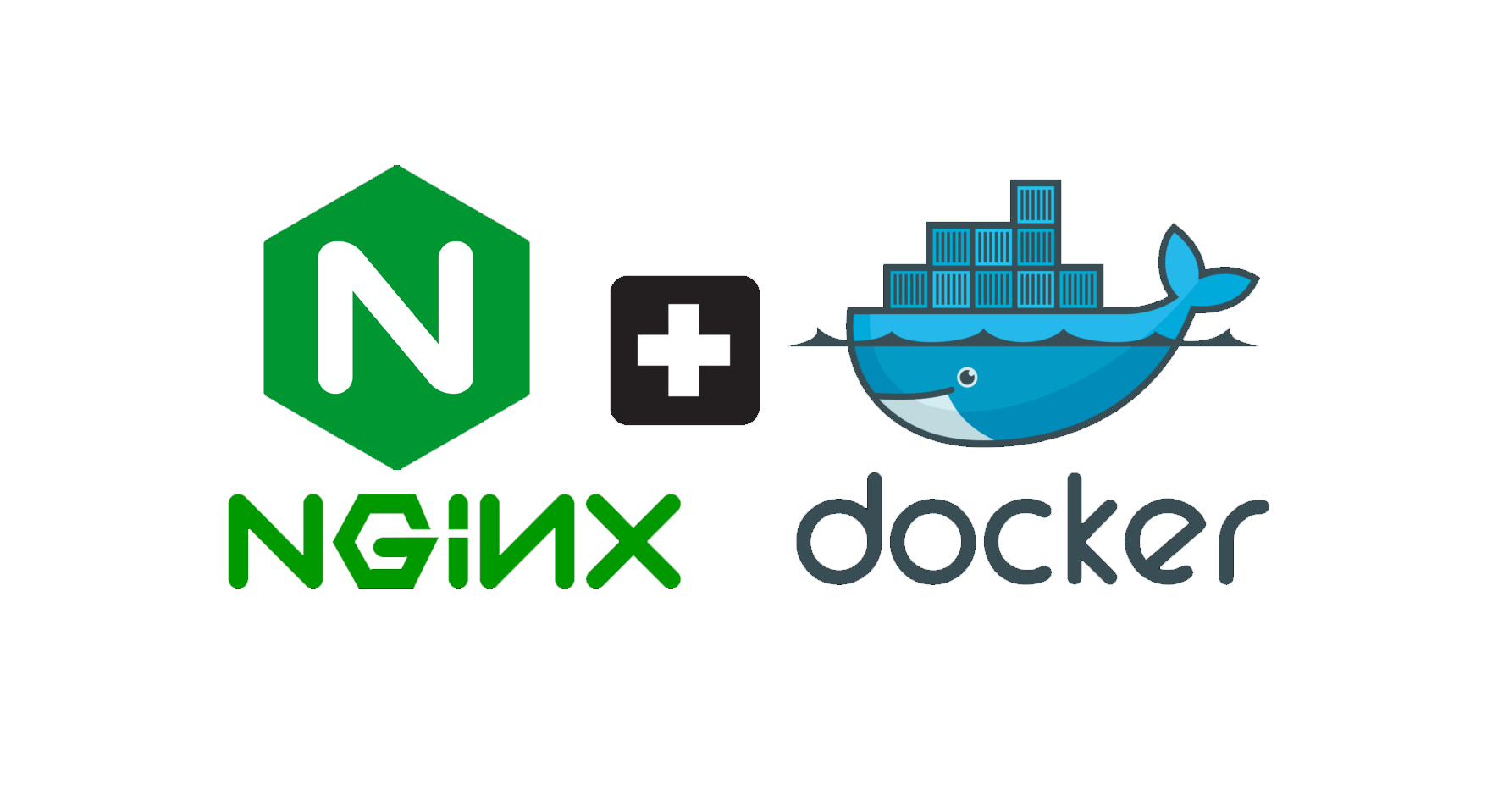
Why Microservices?
Modern applications are increasingly being built using a microservices architecture, where independent services communicate through APIs. This makes them more scalable, maintainable, and deployable. But managing those services locally and in production can be a nightmare without proper tooling.
This is where Docker and NGINX step in. Docker helps you containerize each service, while NGINX can be used as a reverse proxy to route incoming requests appropriately. Together, they provide a lightweight and flexible setup for building your own service-based architecture.
Setting Up the Project
Let’s say you’re building two separate microservices: service-a
and service-b
. Each runs independently, and we use NGINX to unify them under one domain.
Project Structure
├── service-a/
│ ├── Dockerfile
│ └── ...
├── service-b/
│ ├── Dockerfile
│ └── ...
├── nginx.conf
└── docker-compose.yml
docker-compose.yml
This is your orchestration file, which defines how containers are built and networked.
version: '3.8'
services:
service-a:
build: ./service-a
ports:
- "3001:3000"
service-b:
build: ./service-b
ports:
- "3002:3000"
nginx:
image: nginx:latest
ports:
- "80:80"
volumes:
- ./nginx.conf:/etc/nginx/nginx.conf
depends_on:
- service-a
- service-b
nginx.conf
This file tells NGINX how to route requests to each service:
http {
server {
listen 80;
location /service-a/ {
proxy_pass http://service-a:3000/;
}
location /service-b/ {
proxy_pass http://service-b:3000/;
}
}
}
Now, when you access localhost/service-a
or localhost/service-b
, your requests will be routed to the correct container.
Advantages of This Setup
- Isolation: Each service runs in its own container, with its own dependencies and runtime environment.
- Portability: You can ship your app across dev, staging, and production with consistent behavior.
- Scalability: Spin up more instances of a single service without affecting others.
- Ease of Local Testing: Easily test how services interact with each other using just a few Docker commands.
Challenges and Caveats
While this setup is powerful, it comes with some tradeoffs:
- Configuration Complexity: Managing NGINX config and inter-container networking can get tricky as the number of services increases.
- Monitoring Overhead: You’ll need tools like Prometheus, Grafana, or ELK Stack to monitor logs, health, and performance.
- Cold Start Time: Spinning up several containers might slow down your local dev environment, depending on your machine.
Optimizing the Workflow
To keep things manageable as your suite grows:
- Use a
.env
file to store shared port values, secrets, and environment modes. - Implement health checks in your
docker-compose.yml
so services don’t start until dependencies are ready. - Consider using a service mesh like Istio or Traefik if you plan to move into Kubernetes later.
Real-World Use Cases
This architecture is a great foundation for any of the following:
- A dashboard + backend API combo (separate front-end and API containers)
- Auth, billing, and notification services, each in their own container
- A lightweight CMS, analytics engine, and user-facing app running side-by-side
Conclusion
Combining Docker and NGINX gives you a clean, developer-friendly way to build, test, and deploy microservice-based applications. It takes some configuration work upfront, but the benefits in maintainability and scalability are well worth it.
If you're building projects that need to grow modularly, learning this workflow early can save you countless headaches down the line.